Taking Control: Loop Control Statements in Bash Scripting
- compnomics
- Feb 20
- 2 min read
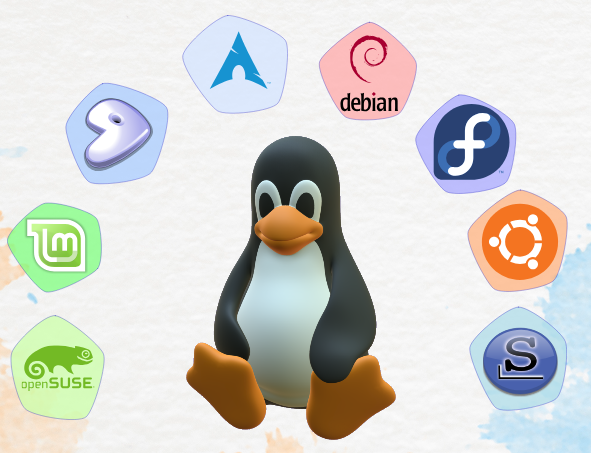
Loops are powerful tools in Bash scripting, allowing you to automate repetitive tasks. But sometimes, you need more control over how a loop behaves. That's where loop control statements come in. Today, we'll explore break and continue with simple examples, showing you how to fine-tune your loops.
1. The break Statement: Exiting a Loop
The break statement immediately terminates the loop, regardless of whether the loop's condition is still true. It's like an emergency exit for your loop.
Example 1: Breaking on a Specific Condition
#!/bin/bash
for i in {1..10}; do
if [ $i -gt 5 ]; then
echo "Breaking the loop at i = $i"
break
fi
echo "i = $i"
done
Explanation:
The loop iterates from 1 to 10.
The if statement checks if i is greater than 5.
If it is, the break statement is executed, and the loop terminates.
Therefore, the loop prints numbers 1 to 5, and then terminates.
Example 2: Breaking in a while loop.
#!/bin/bash
count=1
while true; do
if [ $count -gt 5 ]; then
echo "Breaking the while loop"
break
fi
echo "Count: $count"
count=$((count+1))
done
Explanation:
The while loop uses true, to create an infinite loop.
The if statement checks if count is greater than 5.
If it is, the break statement is executed, and the loop terminates.
2. The continue Statement: Skipping to the Next Iteration
The continue statement skips the rest of the current iteration of the loop and proceeds to the next iteration. It's like saying, "Skip this one, but keep going."
Example 3: Skipping Odd Numbers
#!/bin/bash
for i in {1..10}; do
if [ $((i % 2)) -ne 0 ]; then
continue
fi
echo "Even number: $i"
done
Explanation:
The loop iterates from 1 to 10.
$((i % 2)) calculates the remainder when i is divided by 2.
If the remainder is not 0 (meaning i is odd), the continue statement is executed.
Therefore, only even numbers are printed.
Example 4: Skipping specific files in a for loop.
#!/bin/bash
for file in *.txt; do
if [ "$file" == "skipme.txt" ]; then
echo "Skipping skipme.txt"
continue
fi
echo "Processing file: $file"
# Add your code to process the file here.
done
Explanation:
This loop will iterate through all .txt files in the current folder.
If the filename is "skipme.txt" then the continue command will be called, and the loop will move to the next file.
Example 5: Combining break and continue
#!/bin/bash
for i in {1..10}; do
if [ $i -eq 3 ]; then
continue # Skip 3
elif [ $i -gt 7 ]; then
break # Exit after 7
fi
echo "i = $i"
done
Explanation:
This loop will skip the number 3, and terminate after the number 7 is processed.
Key Takeaways:
break terminates the entire loop.
continue skips the current iteration and proceeds to the next.
These statements give you fine-grained control over your loop's behavior.
Comments