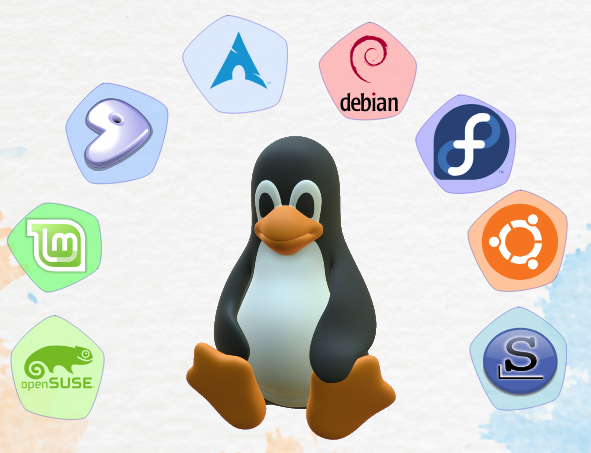
Bash offers powerful substitution mechanisms that allow you to dynamically replace parts of your commands and scripts. This post will explore filename/globbing, variable, command, and arithmetic substitution, providing you with the tools to create more versatile Bash scripts.
Types of Substitution:
Filename/Globbing Substitution:
Expands patterns to match filenames.
Uses wildcards like *, ?, and [].
Example: ls *.txt (lists all .txt files in the current directory).
Variable Substitution:
Replaces a variable with its value.
Uses the dollar sign ($).
Example: echo $HOME (displays the user's home directory).
Command Substitution:
Replaces a command with its output.
Uses backticks (`) or $(...).
Example: echo "Current date: $(date)" (displays the current date).
Arithmetic Substitution:
Performs arithmetic operations.
Uses $((...)).
Example: echo "Sum: $((10 + 5))" (displays the sum of 10 and 5).
Simple Bash Script Example:
#!/bin/bash
# Filename/Globbing Substitution
echo "Text files in current directory:"
ls *.txt
# Variable Substitution
username=$USER
echo "Current user: <span class="math-inline">username"
\# Command Substitution
current\_date\=</span>(date)
echo "Current date: <span class="math-inline">current\_date"
\# Arithmetic Substitution
result\=</span>((20 - 5))
echo "Result of 20 - 5: $result"
#Combine them all
echo "The user $USER, ran this script on $(date), and the result of 10+5 is $((10+5)), inside of the directory $(pwd)"
Practice Session 1: With Example Commands
Create several files with different extensions (e.g., file1.txt, file2.log, data.csv).
touch file1.txt file2.log data.csv
Use filename substitution to list all .txt files.
ls *.txt
Define a variable my_dir with the value of the current directory.
my_dir=$(pwd)
Display the value of my_dir.
echo $my_dir
Use command substitution to display the current time in seconds since the epoch.
echo "Seconds since epoch: $(date +%s)"
Use arithmetic substitution to calculate and display the product of 7 and 8.
echo "Product: $((7 * 8))"
Use all 4 substitutions in one echo command. For example, print the name of all log files, the current user, the date, and the result of 2+2.
echo "Log files: $(ls *.log), user: $USER, date: <span class="math-inline">\(date\), 2\+2\=</span>((2+2))"
Practice Session 2: Without Example Commands
Create a set of files with different extensions in your current directory.
Use filename substitution to list all files with a specific extension.
Define a variable with a string value of your choice.
Display the variable's value within a sentence.
Use command substitution to display the output of the uptime command.
Use arithmetic substitution to calculate and display the result of a mathematical expression.
Combine all four substitution types in a single echo command to display a dynamic message.
Create a variable that contains a file name, and then use globbing to list all files that start with the variable content.
Create a variable that contains a number, and then use arithmetic substitution to multiply that number by 10.
Use command substitution to get the current date, and then use variable substitution to display it in a sentence.
Comments